- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Environmental Science
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- articles and Answers
- Effective Resume Writing
- HR Interview articles
- Computer Glossary
- Who is Who
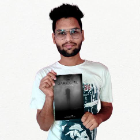
Updated on 09-Apr-2023 11:00:56
Program : To print hello world using C++
// Your First C++ Program
#include<iostream>
int main() {
std::cout << "Hello World!";
return 0;
}
Output :
Hello World!
Explanation:
// Your First C++ Program in C++, any line starting with // is a comment. Comments are intended for the person reading the code to better understand the functionality of the program. It is completely ignored by the C++ compiler.
#include : The #include is a preprocessor directive used to include f... Read Mores
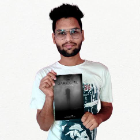
Updated on 20-Dec-2021 20:18:00
Program : Find Smallest Element in Array in C Programming
#include <stdio.h>
int main() {
int a[30], i, num, smallest;
printf("\nEnter no of elements :");
scanf("%d", &num);
//Read n elements in an array
for (i = 0; i < num; i++)
scanf("%d", &a[i]);
//Consider first element as smallest
smallest = a[0];
for (i = 0; i < num; i++) {
if (a[i] < smallest) {
smallest = a[i];
}
}
// Print out t... Read Mores
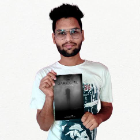
Updated on 20-Dec-2021 20:11:22
Program : Addition of All Elements of the Array
#include <stdio.h>
int main() {
int i, arr[50], sum, num;
printf("\nEnter no of elements :");
scanf("%d", &num);
//Reading values into Array
printf("\nEnter the values :");
for (i = 0; i < num; i++)
scanf("%d", &arr[i]);
//Computation of total
sum = 0;
for (i = 0; i < num; i++)
sum = sum + arr[i];
//Printing of all elements of array
for (i = 0; i < num; i... Read Mores
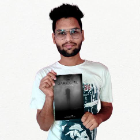
Updated on 19-Dec-2021 17:47:06
Program : To delete duplicate elements in an array
#include <stdio.h>
int main() {
int arr[20], i, j, k, size;
printf("\nEnter array size : ");
scanf("%d", &size);
printf("\nAccept Numbers : ");
for (i = 0; i < size; i++)
scanf("%d", &arr[i]);
printf("\nArray with Unique list : ");
for (i = 0; i < size; i++) {
for (j = i + 1; j < size;) {
if (arr[j] == arr[i]) {
for (k = j; k < size; k++) {
... Read Mores
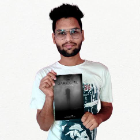
Updated on 19-Dec-2021 17:41:12
#include <stdio.h>
#include <conio.h>
#define MAX 30
void main() {
int size, i, arr[MAX];
int *ptr;
clrscr();
ptr = &arr[0];
printf("\nEnter the size of array : ");
scanf("%d", &size);
printf("\nEnter %d integers into array: ", size);
for (i = 0; i < size; i++) { scanf("%d", ptr); ptr++; } ptr = &arr[size - 1]; printf("\nElements of array in reverse order are :"); for (i = size - 1; i >= 0; i--) {
printf("\nElement... Read Mores
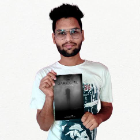
Updated on 18-Dec-2021 18:27:23
Program for implementing a stack using arrays.It involves various operations such as push,pop,stack empty,stack full and display.
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#define size 5
struct stack {
int s[size];
int top;
} st;
int stfull() {
if (st.top >= size - 1)
return 1;
else
return 0;
}
void push(int item) {
st.top++;
st.s[st.top] = item;
}
int stempty() {
if (st.top == -1)
return 1... Read Mores
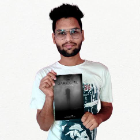
Updated on 16-Dec-2021 16:52:22
This is how we can find the nth highest salary in SQL SERVER using TOP keyword:
SELECT TOP 1 salary FROM ( SELECT DISTINCT TOP N salary FROM Employee ORDER BY salary DESC ) AS temp ORDER BY salary
This is how we can find the nth highest salary in MYSQL using LIMIT keyword:
SELECT salary FROM Employee ORDER BY salary DESC LIMIT N-1, 1
... Read Mores
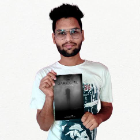
Updated on 16-Dec-2021 16:49:13
To insert multiple rows in SQL we can follow the below syntax:
INSERT INTO table_name (column1, column2,column3...)
VALUES
(value1, value2, value3…..),
(value1, value2, value3….),
...
(value1, value2, value3);
We start off by giving the keywords INSERT INTO then we give the name of the table into which we would want to insert the values. We will follow it up with the list of the columns, for which we would have to add the values. Then we will give in the VALUES key... Read Mores
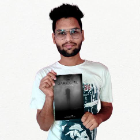
Updated on 16-Dec-2021 16:46:22
A trigger is a stored program in a database which automatically gives responses to an event of DML operations done by insert, update, or delete. In other words, is nothing but an auditor of events happening across all database tables.
Let’s look at an example of a trigger :
CREATE TRIGGER bank_trans_hv_alert
BEFORE UPDATE ON bank_account_transaction
FOR EACH ROW
begin
if( abs(:new.transaction_amount)>999999)THEN
RAISE_APPLICATION_ERROR(-20000, 'Account transaction exceedi... Read Mores
Advertisements
ads